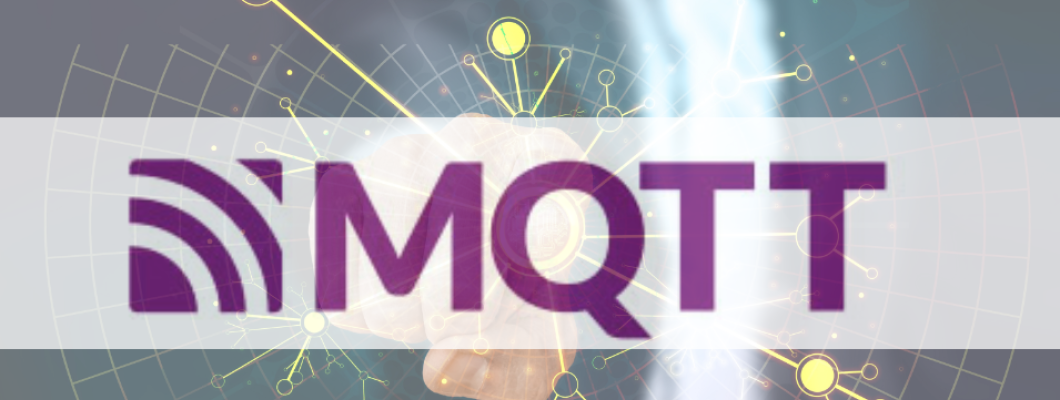
MQTT protocol - MQTT Development in IoT and Beyond
In the era of the Internet of Things (IoT), efficient communication between devices is paramount. As the number of connected devices increases, so does the need for lightweight, scalable and reliable protocols that can manage data exchange. One protocol that has gained prominence due to its effectiveness in these environments is MQTT protocol (Message Queuing Telemetry Transport).
Originally developed for monitoring oil pipelines via satellite links,MQTT protocol has become the de facto standard for IoT communications, enabling devices to communicate with minimal bandwidth and low power consumption. Below you will find a comprehensive guide after using MQTT protocol, from understanding its architecture and basic concepts to practical implementation and advanced features.
What is MQTT? Understanding the Basics of MQTT
MQTT is a lightweight publish-subscribe network protocol that transmits messages between devices. It is designed to be simple and easy to implement, making it ideal for resource-constrained environments such as IoT devices with low processing power and limited bandwidth.
Model publish-subscribe: Unlike traditional models request-response, MQTT protocol uses a publish-subscribe model. This means that devices (clients) can publish messages to a topic, and other devices can subscribe to it this topic to receive messages. This separates the message sender (publisher) from the recipients (subscribers), which leads to more flexible communication.
Broker: The broker is the central hub in the MQTT network. It receives messages from publishers and forwards them to subscribers. The broker handles all message routing and filtering based on topics.
Basic Components of the MQTT Protocol
Understanding the core components of the MQTT protocol is essential for effective protocol implementation:
Customer: Any device or application that connects to an MQTT broker. Clients can post messages, subscribe to topics, or do both.
Broker: Server managing all routing and message delivery. It is responsible for receiving messages from publishers and sending them to the appropriate subscribers.
Subject: A string that the broker uses to filter messages for each connected client. Topics are hierarchical, allowing for granular message routing.< /span>
Message: Data transferred between clients. Each message has a subject, payload (actual data), quality of service (QoS) level, and other optional parameters.
Getting Started with the Protocol MQTT: Configuring the MQTT Protocol Environment
Before starting the MQTT implementation, it is important to configure the environment correctly. This includes selecting the appropriate MQTT broker, installing the necessary software, and configuring the network.
MQTT Broker Selection
The broker is a key part of any MQTT setup as it handles all message routing. There are several popular brokers available, each with their own features and benefits:
Mosquitto: Mosquitto is an open source MQTT broker that is widely used due to its light weight and easy configuration. It is suitable for both small IoT projects and large corporate deployments.
HiveMQ: HiveMQ to commercial MQTT broker designed for high availability and scalability. It is ideal for enterprise environments where reliability and performance are critical.
EMQX: EMQX is another open-source MQTT broker that focuses on scalability and handling millions of concurrent connections. It is suitable for large-scale IoT applications.
Installing and Configuring the MQTT Broker
Here's how to install and configure Mosquitto, one of the most popular MQTT brokers:
Mosquitto Installation:
On Ubuntu, you can install Mosquitto with the following commands:< /p>
bash
sudo apt-add-repository ppa:mosquitto-dev/mosquitto-ppa< /span>
sudo apt-get update
sudo apt-get install mosquitto mosquitto-clients
For Windows, the installer can be downloaded from the official Mosquitto website.
The main configuration file is in /etc/mosquitto/mosquitto.conf (on Linux). You can edit this file to customize settings such as port, authentication, and logging.
For basic use, the default configuration is usually sufficient. However, you may want to configure authentication and access controls for additional security.
On Linux, start the broker with the following command:
On Windows, you can run Mosquitto from the Start menu or from the command line.
To test the broker, you can use the mosquitto_pub and mosquitto_sub command line tools to publish and subscribe to topics. For example:
Paho MQTT: The Eclipse Paho project provides client libraries MQTT for various programming languages including Python, Java and JavaScript.
MQTT.fx: A popular GUI tool for testing and debugging MQTT messages. It is useful for developers who want to have a visual interface to interact with their MQTT broker.
Node-RED: A flow-based development tool that includes built-in MQTT nodes, enabling rapid development and deployment of IoT applications.
Installing Paho MQTT:
Install the Paho MQTT client library for Python using pip:
Here is a basic Python script to publish a message:
Run the script from the terminal or command line:
This will post a "Hello MQTT" message to the "test/topic" topic on your broker.
Writing a subscriber script:
Here is a Python script to subscribe to a topic:
Run script:
The subscriber will print all messages that he receives with the subject "test/subject".
QoS 0: At most once:
The message is delivered at most once, without an acknowledgment of receipt. This is the fastest and most effective QoS level, but there is a risk that messages may be lost.
Example use case: non-critical sensor data.
QoS 1: At least once:
The message is delivered at least once, with confirmation from the recipient required. This ensures that the message is received, but may result in duplicate messages.
Example use case: billing or transaction data.
QoS 2: Exactly once:
The message is delivered exactly once, with a comprehensive handshake between the sender and the recipient. This is the most reliable, but also the slowest, level of QoS.
Example use case: critical system alerts.
Setting QoS in Python:
You can set the QoS level when posting or subscribing to a topic. For example:
Publish a saved message:
You can publish a saved message by setting the save flag to True:
Use case:
Stored messages are especially useful in situations where you need to immediately provide new subscribers with information about the current status of a device or sensor.
Configuring LWT:
You can set the LWT message when creating the MQTT client:
Use case:
LWT technology is useful in situations where you need to monitor the status of devices and receive notifications if any of them go into standby mode. offline.
Single-level wildcard (+):
The + wildcard matches one level in the topic hierarchy. For example, the subscription home/+/temperature would match both home/livingroom/temperature and home/kitchen/temperature.
Multi-level symbol (#):
The # wildcard matches all other levels in the topic hierarchy. For example, the home/# subscription would match home/livingroom/temperature, home/kitchen/humidity and other subtopics in home/.
Using wildcards:
Wildcards can be used when subscribing to topics. For example:
Use case:
Wildcards are especially useful in situations where you have a large number of similar devices and you want to receive messages from all of them within one subscription.
Configuring a persistent session:
You can create a persistent session by setting the clean_session flag to False when establishing a connection:
Use case:
Persistent sessions are useful in situations where you want to make sure that no messages are lost even if a client temporarily disconnects.
Configuring the bridge:
The bridge configuration is typically done in the broker configuration file. For example, in Mosquitto you can add a bridge configuration like this:
Use case:
The bridging feature is useful in situations where you need to connect different geographic locations or scale MQTT network across multiple brokers.
Enable TLS:
To enable TLS, you need to configure your broker with the appropriate certificates. For example in Mosquitto:
Clients must also be configured to use TLS:
TLS is crucial in situations where sensitive data is transmitted, such as in healthcare applications or finances.
In Mosquitto, you can enable username and password authentication by creating a password file :
Then update the configuration file:
Use case:
< ul style="margin-top:0;margin-bottom:0;padding-inline-start:48px;">Authentication is necessary in situations where access control is critical, such as in multi-user environments or when processing sensitive data.
Configuring access control lists:
In Mosquitto you can create an ACL file defining permissions for each user:
Update config file to use access control list:
Use case:
ACLs are useful in situations where strict access controls are required, such as in enterprise environments or public brokers MQTT.
Configuring Mosquitto:
Broker launch:
sudo systemctl start mosquitto
Testing the broker:
mosquitto_pub -t "test/topic" -m "Hello, MQTT"
MQTT Client Selection
After configuring the broker, you need to select the MQTT clients that will publish and subscribe to topics. MQTT clients are available for a wide range of platforms, including:
Implementing the MQTT protocol step by step
Now that your environment is set up, it's time to implement MQTT in a real-life scenario. This section will walk you through creating a basic MQTT application, including publishing messages, subscribing to topics, and managing quality of service (QoS).
Publishing Messages
Posting messages is a core function of MQTT. Here's how you can post a message using Python and the Paho MQTT client:
pip install paho-mqtt
2. Writing the publisher script:
import paho.mqtt.client as mqtt
# Define the MQTT broker and port
broker = "localhost"
port = 1883# Create a new MQTT client instance
client = mqtt.Client()
# Connect to the broker
.connect(broker, port)# Publish a message to the topic "test/topic"
client.publish("test/topic", "Hello, MQTT ")
# Disconnect from the broker
client.disconnect()3. Starting Publishers:python mqtt_publisher.py
B. Subscribing to Topics
Subscribing to topics allows MQTT clients to receive messages published in those topics. Here's how to write a basic subscriber in Python:
import paho.mqtt .client as mqtt
# Define the MQTT broker and port
broker = "localhost"
port = 1883# Define the callback function for receiving messages
def on_message(client, userdata, message):
print(f"Received message: {message.payload.decode( )} on topic {message.topic}")
# Create a new MQTT client instance
client = mqtt.Client()
# Assign the on_message callback function
client.on_message = on_message
# Connect to the broker
client.connect(broker, port)# Subscribe to the topic "test/topic"
client.subscribe("test/topic")
# Start the loop to process incoming messages
client.loop_forever()
2. Start Subscriber:
python mqtt_subscriber.py
Understanding Quality of Service (QoS)
MQTT supports three levels of quality of service (QoS) that define how messages are delivered between clients and brokers:
client.publish("test/topic", "Hello, MQTT", qos=1 )
client.subscribe("test/topic", qos=2)
Advanced MQTT Features - Improving MQTT implementation
Once you get the hang of the basics, you can start exploring some of the more advanced features which gives MQTT protocol. These functions enable more sophisticated and robust implementations.
MQTT Preserved Messages
A stored message in MQTT is a message that is stored by the broker and sent to all new subscribers who subscribecrypt topic. This is useful to ensure that new subscribers receive the latest status immediately after subscribing.
client.publish("test/topic", "Hello, MQTT", retain=True)
LWT function
The Last Will and Testament (LWT) feature in MQTT allows you to define a message that will be published by the broker if a client disconnects unexpectedly. This is useful for detecting and handling client failures.
client.will_set("test/status", "Client disconnected unexpectedly ", qos=1, retain=True)
Theme wildcards
The MQTT protocol supports topic wildcards, which allow multiple topics to be subscribed to with a single subscription. There are two types of wildcards:< /span>
client.subscribe("home/+/temperature")
client.subscribe("home/#")
Permanent sessions
In MQTT, a persistent session allows the broker to store information about a client's subscription and undelivered messages. When the client reconnects, it will receive all messages that were posted while it was offline.
client.connect(broker, port, clean_session=False)
Connecting MQTT brokers
Bridging allows multiple MQTT brokers to be connected, allowing messages to be shared across different networks or locations. This is especially useful for large-scale deployments where multiple brokers are required.
connection bridge-to-remote
address remote-broker.example.com:1883
topic # both 0
Security best practices for MQTT
Even though MQTT protocol is designed to be lightweight, it is necessary to implement security measures to protect the MQTT network from unauthorized access and data breaches .
Transport layer security (TLS)
TLS is a protocol that provides encryption and secure communication over the network. The TLS implementation in MQTT ensures that all data transferred between clients and the broker is encrypted.
listener 8883
cafile /etc/mosquitto/certs/ca.crt
certfile /etc/mosquitto/certs/server.crt
keyfile /etc/mosquitto/certs/server.key
client.tls_set(ca_certs="ca.crt", certfile= "client.crt", keyfile="client.key")
Use case:
Authentication and authorization
MQTT brokers can be configured to require clients to authenticate before connecting. Authentication can be done using usernames and passwords or more advanced methods such as certificates.
Set up authentication:
sudo mosquitto_passwd -c /etc/mosquitto/passwd username
allow_anonymous false
password_file /etc/mosquitto/passwd
Access Control Lists (ACL)
Access Control Lists (ACLs) allow you to define the topics a client can publish to or subscribe to, providing fine-grained access control.pu to the MQTT network.
user client1
topic read home/temperature
topic write home/temperature
acl_file /etc/mosquitto/aclfile
The future of the MQTT protocol
MQTT has proven to be a robust and versatile protocol for IoT and beyond. Its lightweight nature combined with features such as QoS, persistent sessions, and flexible topic management make it ideal for a wide range of applications, from smart homes to industrial automation.
As the IoT evolves, the role of MQTT is set to expand even further, with new developments such as MQTT-SN (MQTT for Sensor Networks) and improved integration with cloud platforms. However, as with any technology, it is crucial to implement best practices, especially in the area of security, to ensure that MQTT implementations are both effective and secure.
Mastering Using MQTT, you can unlock the full potential of IoT and create efficient, scalable, and reliable systems. Whether you are a developer, systems architect, or IoT enthusiast, understanding and leveraging the opportunities of MQTT protocol will be essential as we move into a more connected world.